普通宏
#include <stdio.h>
#define MAX(x, y) x > y ? x : y
int main() {
int x = 3;
int y = 4;
int z = MAX(x, y);
printf("%d\n", z);
return 0;
}
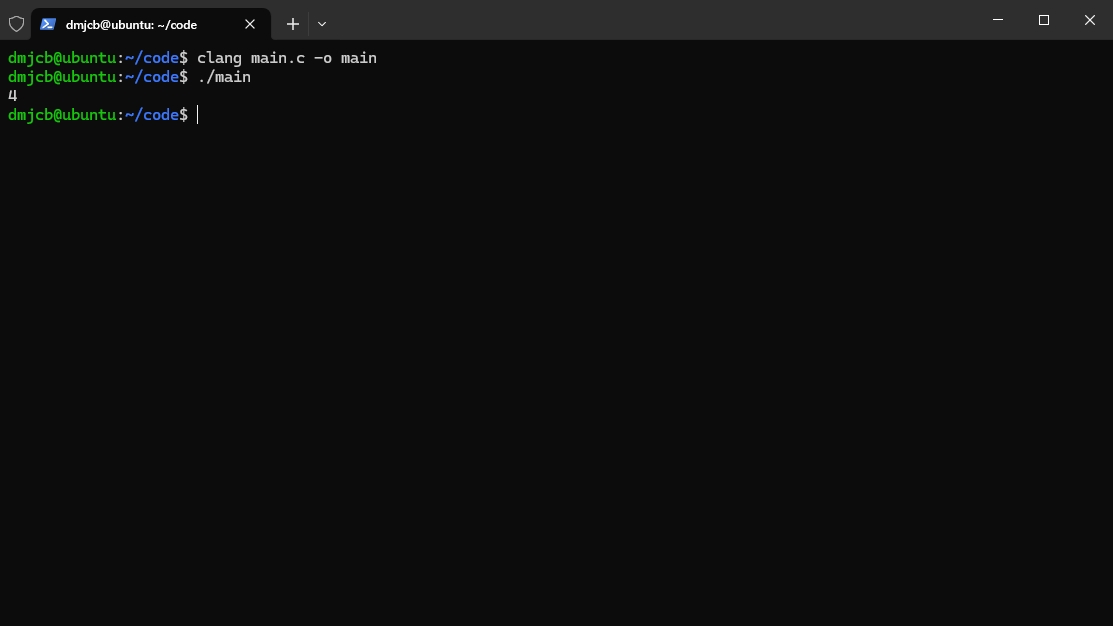
内置宏
预处理
指令 |
说明 |
# |
空指令, 无任何效果 |
#include |
包含一个源代码文件 |
#define |
定义宏 |
#undef |
取消已定义宏 |
#if |
若给定条件为真,则编译下面代码 |
#ifdef
#if defined |
若宏已经定义,则编译下面代码 |
#ifndef |
若宏没有定义,则编译下面代码 |
#elif |
若前面#if 给定条件不为真, 且当前条件为真 |
#endif |
结束一个#if …#else 条件编译块 |
#ifndef __INCLUDE_HPP__
#define __INCLUDE_HPP__
// ...
#endif
#define FLAG
// 等价于 #ifdef FLAG
#if defined(FLAG)
// ...
#endif
#undef FLAG
预定义
系统
宏名 |
说明 |
_WIN32 |
在所有wndows系统(32/64位)上都定义 |
_WIN64 |
仅在64位windows系统上定义 |
__linux__ |
在linux系统上定义 |
__unix__ |
在unix系统上定义 |
__APPLE__ |
在apple系统上定义 |
__ANDROID__ |
在安卓 系统上定义 |
#include <iostream>
int main() {
#if defined(_WIN64)
std::cout << "Windows 64" << std::endl;
#elif defined(__linux__)
std::cout << "Linux" << std::endl;
#elif defined(__unix__)
std::cout << "Unix" << std::endl;
#elif defined(__APPLE__)
std::cout << "Apple" << std::endl;
#elif defined(__ANDROID__)
std::cout << "Android" << std::endl;
#endif
return 0;
}
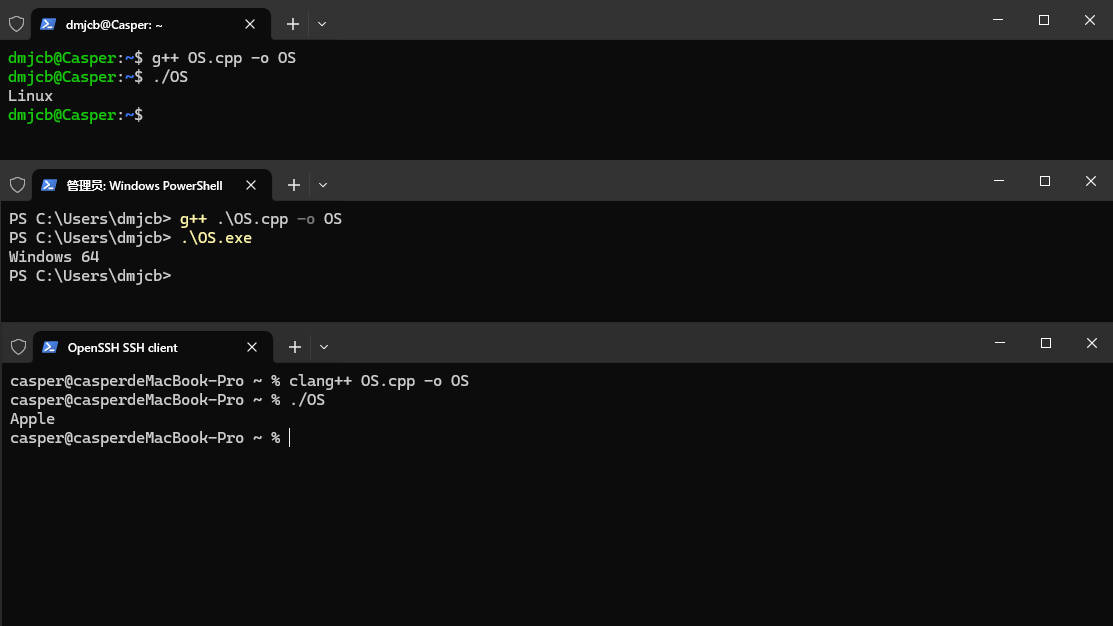
C++版本
__cplusplus
是C++编译器特有宏, 只在C++编译器中定义, 显示当前支持C++版本
值 |
说明 |
199711L |
C++98/03 |
201103L |
C++11 |
201402L |
C++14 |
201703L |
C++17 |
202002L |
C++20 |
#include <iostream>
int main() {
#if __cplusplus >= 202002L
std::cout << "C++20 or newer\n";
#elif __cplusplus >= 201703L
std::cout << "C++17\n";
#elif __cplusplus >= 201402L
std::cout << "C++14\n";
#elif __cplusplus >= 201103L
std::cout << "C++11\n";
#else
std::cout << "C++98/03 or older\n";
#endif
return 0;
}
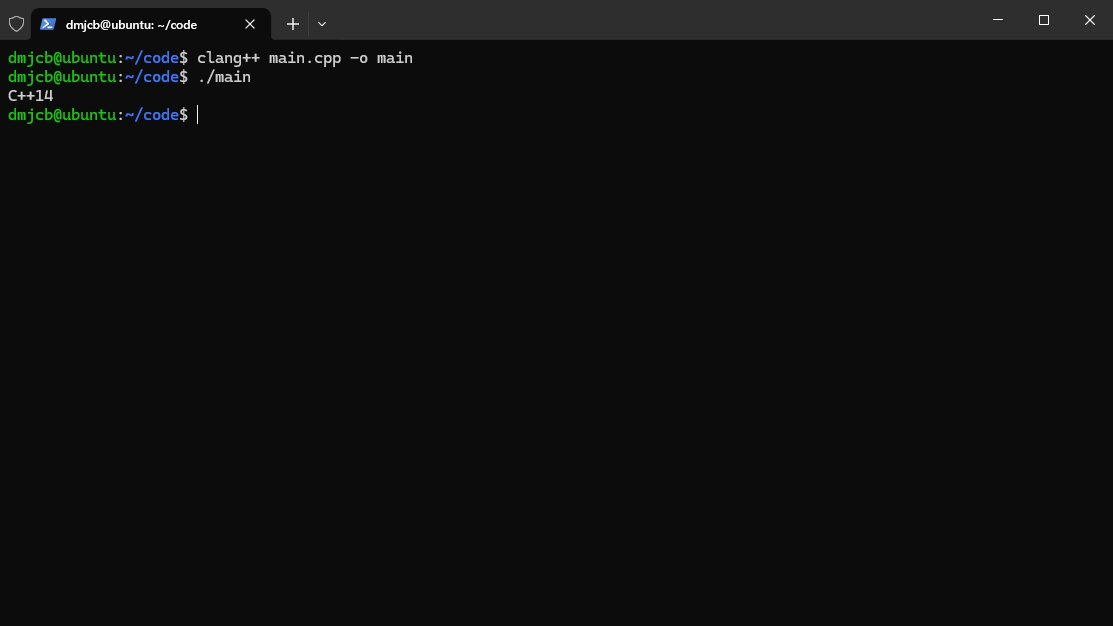
功能宏
宏 |
说明 |
__LINE__ |
表示当前行号, 整型 |
__FILE__ |
表示当前文件名, 字符串类型 |
__DATE__ |
编译日期, 字符串类型 |
__TIME__ |
编译时间, 字符串类型 |
__FUNCTION__ |
当前函数名 |
#include <iostream>
void Hello() {
std::cout << "file: " << __FILE__ << std::endl;
std::cout << "line: " << __LINE__ << std::endl;
std::cout << "date: " << __DATE__ << std::endl;
std::cout << "time: " << __TIME__ << std::endl;
std::cout << "func: " << __FUNCTION__ << std::endl;
}
int main() {
Hello();
return 0;
}
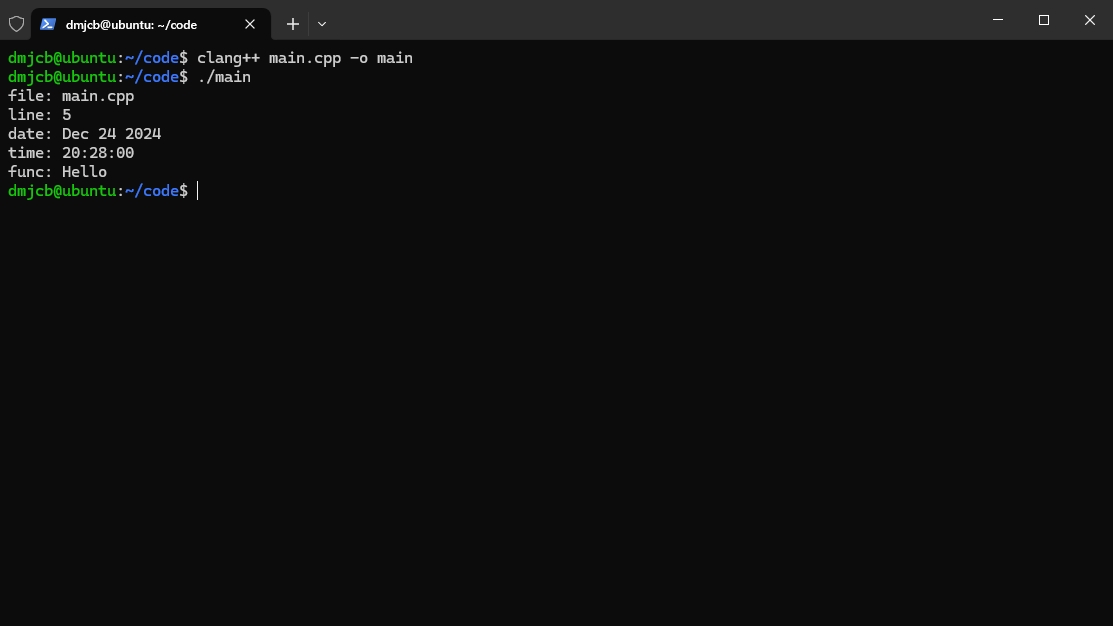